How to Design Custom Flutter Buttons
While Flutter offers a variety of built-in button widgets, you may want to create custom buttons that match your app's unique design. In this blog post, we will walk through the steps to design custom Flutter buttons.
Share this Post to earn Money ( Upto ₹100 per 1000 Views )
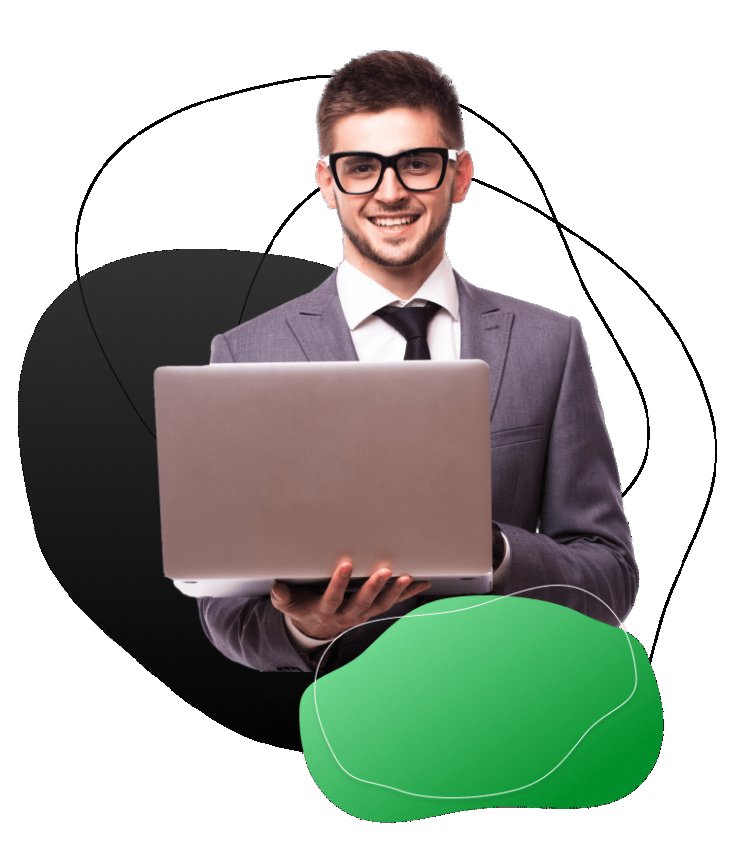
Flutter provides a flexible and powerful framework for designing user interfaces, including buttons. While Flutter offers a variety of built-in button widgets, you may want to create custom buttons that match your app's unique design. In this blog post, we will walk through the steps to design custom Flutter buttons.
Step 1: Define the Button Design
The first step in designing a custom Flutter button is to define its visual appearance. Consider the following aspects:
Button Shape: Decide whether you want a rectangular, circular, or any other shape for your button.
Button Size: Determine the dimensions of your button, such as width and height.
Button Colors: Choose the background color, text color, and any other colors you want to use for your button.
Button Typography: Select the font style, size, and weight for the button text.
Step 2: Create a Custom Button Widget
Once you have defined the design of your custom button, you can create a custom widget to encapsulate its functionality and appearance. Here's an example of how you can create a custom button widget:
import 'package:flutter/material.dart';
class CustomButton extends StatelessWidget {
final String text;
final VoidCallback onPressed;
const CustomButton({required this.text, required this.onPressed});
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: onPressed,
child: Text(
text,
style: TextStyle(
fontSize: 16,
fontWeight: FontWeight.bold,
),
),
style: ElevatedButton.styleFrom(
primary: Colors.blue, // Set the background color of the button
onPrimary: Colors.white, // Set the text color of the button
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(8), // Set the button shape
),
),
);
}
}
In this example, we create a CustomButton widget that extends the StatelessWidget class. The widget takes two required parameters: text for the button label and onPressed for the button's callback function.
Step 3: Implement the Custom Button
To use the custom button in your Flutter app, follow these steps:
Import the custom button widget into your Dart file.
Add an instance of the CustomButton widget to your app's widget tree.
Provide the necessary parameters, such as the button text and the callback function.
Here's an example of how you can implement the custom button in your app:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Custom Button Example'),
),
body: Center(
child: CustomButton(
text: 'Click Me',
onPressed: () {
// Add your button's functionality here
print('Button clicked!');
},
),
),
),
);
}
}
In this example, we create a simple Flutter app with a custom button. When the button is clicked, it prints a message to the console.
Conclusion
Designing custom Flutter buttons allows you to create buttons that align with your app's unique design requirements. By following the steps outlined in this blog post, you can easily create and implement custom buttons in your Flutter app.
I hope this blog post helps you in designing custom Flutter buttons. Happy coding!