.NET Core Blazor: Feels Illegal to Know
Discover the power of .NET Core Blazor in this comprehensive guide. Learn about its features, setup, components, and best practices for building dynamic web apps.
Share this Post to earn Money ( Upto ₹100 per 1000 Views )
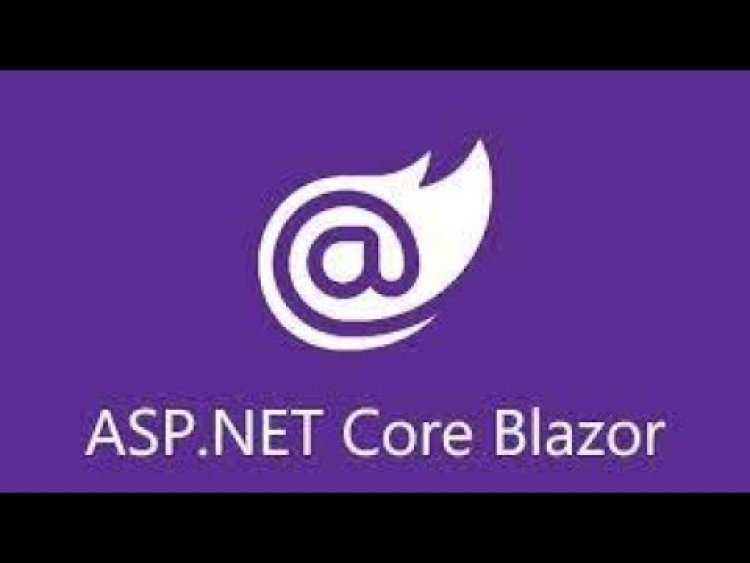
Blazor, part of the ASP.NET Core framework, is a powerful tool for building interactive web applications using C# instead of JavaScript. It allows developers to create rich, dynamic web applications with the full power of .NET. By using Blazor, you can leverage existing .NET libraries and the power of C# to build client-side web applications, making it a popular choice for many developers and a custom net development company.
Blazor offers two hosting models: Blazor Server and Blazor WebAssembly. Blazor Server runs on the server and updates the client-side via SignalR, while Blazor WebAssembly runs entirely on the client’s browser, providing a more interactive experience. Understanding these hosting models helps in deciding the best fit for your project's needs.
Why Choose Blazor for Web Development
Blazor .net core provides numerous advantages for web development, making it a compelling choice:
-
Full-Stack Development with C#: Developers can use C# for both server-side and client-side development, reducing the need for switching languages.
-
Component-Based Architecture: Blazor’s component-based architecture allows for building reusable components, enhancing code maintainability and scalability.
-
Seamless Integration with .NET Libraries: Leverage existing .NET libraries and ecosystem, reducing development time and increasing efficiency.
-
Enhanced Performance: Blazor WebAssembly offers near-native performance by running directly in the browser’s WebAssembly.
When comparing Blazor to other frameworks like Angular, React, or Vue.js, one standout feature is its integration with the .NET ecosystem. This integration provides a seamless development experience, especially for teams already familiar with .NET technologies.
Setting Up Your Development Environment
To start with Blazor .NET Core, you need to set up your development environment. Here are the prerequisites:
-
.NET Core SDK: Install the latest version from the .NET website.
-
Visual Studio: Download and install Visual Studio with the ASP.NET and web development workload.
Installing .NET Core SDK
Begin by downloading the .NET Core SDK from the official website. Follow the installation instructions specific to your operating system. This SDK includes everything you need to build and run .NET applications.
Setting Up Visual Studio
Visual Studio is a powerful integrated development environment (IDE) that supports Blazor development. Ensure you have the latest version installed. During installation, select the ASP.NET and web development workload to get all the necessary tools.
Creating Your First Blazor Application
Creating a Blazor application is straightforward. Follow these steps to create and run your first Blazor project:
-
Open Visual Studio: Start Visual Studio and select "Create a new project."
-
Select Blazor App: Choose the Blazor App template and click "Next."
-
Configure Project: Name your project, choose a location, and select Blazor WebAssembly or Blazor Server as the hosting model.
-
Create Project: Click "Create" to generate the project.
Project Structure
Understanding the default project structure is crucial. A typical Blazor project includes:
-
Pages: Contains Razor components, each representing a page in your application.
-
Shared: Contains shared components used across different pages.
-
wwwroot: Contains static assets like CSS, JavaScript, and images.
Running the Default Application
Once the project is created, press F5 to run the default Blazor application. This action will build the project, start a development server, and open your application in a web browser.
Understanding the Blazor Project Files
Blazor projects consist of various files that play specific roles:
-
_Imports.razor: Contains common namespaces used across components.
-
App.razor: Sets up routing for the application.
-
MainLayout.razor: Defines the layout for the application.
-
Index.razor: Represents the home page.
Components in Blazor
Components are the building blocks of Blazor applications. They encapsulate UI and logic, making them reusable and maintainable.
Building Reusable Components
To create a reusable component:
-
Add New Razor Component: Right-click the Pages folder, add a new Razor component, and name it.
-
Define Markup and Logic: Use HTML and C# to define the component's UI and behavior.
-
Use the Component: Include the component in other pages by referencing its name.
Component Lifecycle
Understanding the component lifecycle helps in managing state and behavior:
-
OnInitialized: Called when the component is initialized.
-
OnParametersSet: Called when parameters are set or changed.
-
OnAfterRender: Called after the component has rendered.
Parameter Passing Between Components
Passing parameters between components is simple:
// ParentComponent.razor
<ChildComponent Title="Hello World" />
// ChildComponent.razor
<h3>@Title</h3>
@code {
[Parameter]
public string Title { get; set; }
}
Data Binding and Event Handling
Data binding and event handling are essential for interactive web applications.
One-way and Two-way Data Binding
Blazor supports one-way and two-way data binding:
-
One-way Binding: Binds data from the model to the UI.
-
Two-way Binding: Binds data both ways, from the model to the UI and vice versa.
<input @bind="Name" />
<p>@Name</p>
@code {
private string Name { get; set; }
}
Handling User Events
Handle user events using C# event handlers:
<button @onclick="HandleClick">Click me</button>
@code {
private void HandleClick()
{
// Event handling logic
}
}
Form Validation in Blazor
Blazor provides built-in support for form validation using data annotations:
<EditForm Model="@user" OnValidSubmit="HandleValidSubmit">
<DataAnnotationsValidator />
<ValidationSummary />
<InputText id="name" @bind-Value="user.Name" />
<button type="submit">Submit</button>
</EditForm>
@code {
private User user = new User();
private void HandleValidSubmit()
{
// Form submission logic
}
}
public class User
{
[Required]
public string Name { get; set; }
}
Blazor Routing and Navigation
Blazor’s routing system enables navigation between components.
Defining Routes
Define routes using the @page directive:
@page "/home"
<h3>Home Page</h3>
Navigating Between Components
Use the NavLink component for navigation:
<NavLink href="/home">Home</NavLink>
<NavLink href="/about">About</NavLink>
Route Parameters
Pass parameters through the URL:
@page "/product/{Id}"
<h3>Product @Id</h3>
@code {
[Parameter]
public string Id { get; set; }
}
Dependency Injection in Blazor
Blazor’s dependency injection (DI) system simplifies service management.
Understanding Dependency Injection
DI is a design pattern used to implement IoC, allowing the creation of dependent objects outside of a class.
Registering Services
Register services in Startup.cs:
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IDataService, DataService>();
}
Injecting Services into Components
Inject services using the @inject directive:
@inject IDataService DataService
<p>@DataService.GetData()</p>
Blazor and JavaScript Interoperability
Blazor .NET Core provides seamless integration with JavaScript, allowing you to call JavaScript functions from Blazor and vice versa.
Calling JavaScript Functions from Blazor
Use the IJSRuntime service to call JavaScript functions:
@inject IJSRuntime JS
<button @onclick="CallJsFunction">Call JavaScript</button>
@code {
private async Task CallJsFunction()
{
await JS.InvokeVoidAsync("alert", "Hello from Blazor!");
}
}
Invoking Blazor Methods from JavaScript
Define JavaScript functions that call Blazor methods:
function callBlazorMethod() {
DotNet.invokeMethodAsync('BlazorApp', 'BlazorMethod');
}
Handling JavaScript Libraries
Integrate popular JavaScript libraries with Blazor to enhance functionality.
State Management in Blazor
State management is crucial for maintaining application consistency.
Understanding State Management
State management involves maintaining the state of an application across different components and user interactions.
Using Local Storage and Session Storage
Blazor provides mechanisms for using local storage and session storage to preserve state.
Implementing State Management Patterns
Implement common state management patterns like MVVM or Redux to handle complex state logic effectively.
Deploying Blazor Applications
Deploying Blazor applications involves publishing the application and choosing the right hosting environment.
Publishing a Blazor Application
Publish your Blazor application using Visual Studio’s publish wizard.
Deployment Options
Choose from various deployment options, including Azure, Docker, or traditional web servers.
Hosting on Azure
Azure provides seamless integration with Blazor applications, offering various hosting plans and services.
Best Practices for Blazor Development
Following best practices ensures your Blazor application is robust, maintainable, and secure.
Performance Optimization Tips
Optimize performance by minimizing JavaScript interop, using asynchronous methods, and leveraging caching.
Security Considerations
Ensure security by following best practices like input validation, secure data storage, and using HTTPS.
Code Organization and Maintainability
Organize code into manageable components and services, use consistent naming conventions, and maintain proper documentation.
Conclusion
Blazor .NET Core is a revolutionary framework that simplifies web development by enabling full-stack development with C#. Its integration with the .NET ecosystem, component-based architecture, and rich feature set make it an excellent choice for modern web applications. Whether you are part of a custom net development company or looking to hire .net developers for your project, Blazor provides a robust platform to build dynamic, high-performance web applications. As Blazor continues to evolve, it promises to bring even more exciting features and improvements, making it an indispensable tool in the web development landscape.