Master Stock Market Insights with Python Stock API
Besides real-time updates, many stock market API services provide historical data. This allows you to analyze trends over time, track price movements, and make predictions based on past performance.
Share this Post to earn Money ( Upto ₹100 per 1000 Views )
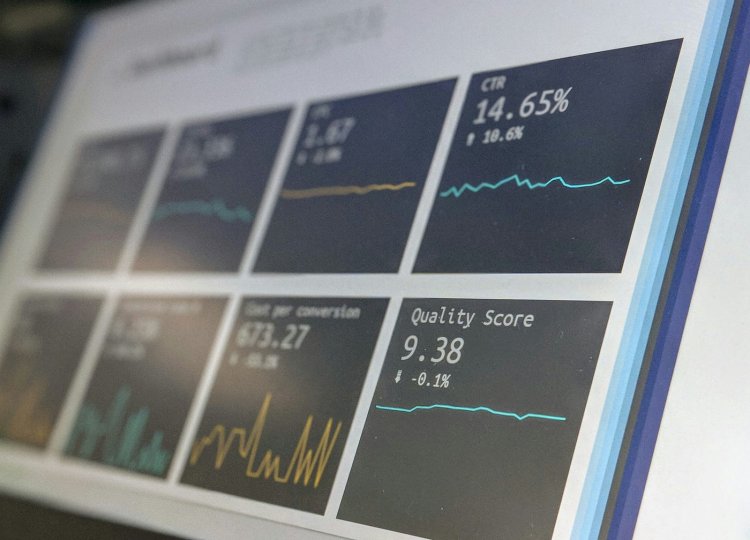
The Ultimate Python Guide for Stock API Integration
If you want to dive into stock market data analysis, Python is one of the best tools you can use. Thanks to stock API Python, you can easily access real-time data, historical prices, and market trends, all from the comfort of your Python environment. In this ultimate guide, we’ll walk you through everything you need to know about integrating a stock market API Python into your projects.
What is a Stock API Python?
Simply put, a stock API is a way to pull stock data directly into your Python scripts. APIs like Alpha Vantage, Yahoo Finance, and IEX Cloud provide a connection to live stock market data, allowing you to easily fetch prices, market performance, and historical data. The real power of these APIs lies in their ability to automate and streamline your data collection and analysis processes.
Using a stock market API means you can gather all sorts of data — from minute-by-minute stock prices to long-term market trends — without having to manually check the stock market every day.
Why Should You Use a Stock Market API Python?
1. Access Real-Time Data
With a stock API, you can get up-to-the-minute stock prices. Whether you’re tracking your investments or building a stock monitoring app, real-time data is essential for making quick decisions.
2. Analyze Historical Data
Besides real-time updates, many stock market API services provide historical data. This allows you to analyze trends over time, track price movements, and make predictions based on past performance.
3. Automate Your Data Collection
Instead of manually searching for stock prices, a stock API Python automates the process. With just a few lines of code, you can fetch data at regular intervals, making your stock analysis much more efficient.
4. Customization
With a stock market API, you can pull exactly the data you need. Whether you’re interested in a specific stock or a particular market index, APIs let you tailor the information to suit your needs.
How to Use a Stock Market API Python: A Step-by-Step Guide
Step 1: Install Required Libraries
Before we start coding, you’ll need to install two essential libraries: requests for making API calls and pandas for analyzing the data.
Run this command to install them:
bash
Copy code
pip install requests pandas
Step 2: Choose a Stock API
There are several stock API providers available. For this example, we’ll use Alpha Vantage. It’s a great API that offers a free plan, and it’s easy to integrate with Python. To get started, sign up for an API key from the
Step 3: Fetch Data from the API
Once you have your API key, it’s time to write some Python code. Here’s an example of how to fetch data for Apple stock (AAPL) using
Copy code
import requests
import pandas as pd
API_KEY = 'your_api_key_here'
symbol = 'AAPL' # Stock symbol for Apple
url = f'https://www.alphavantage.co/query?function=TIME_SERIES_INTRADAY&symbol={symbol}&interval=5min&apikey={API_KEY}'
response = requests.get(url)
data = response.json()
# Convert the data to a DataFrame
time_series = data['Time Series (5min)']
df = pd.DataFrame(time_series).T
# Convert the date column to datetime format
df.index = pd.to_datetime(df.index)
# Display the data
print(df.head())
Step 4: Analyze the Data
Once you’ve fetched the data, it’s time to analyze it. Here’s an example of how to calculate a moving average for the stock price:
python
Copy code
# Calculate the 10-period moving average
df['10_period_ma'] = df['4. close'].astype(float).rolling(window=10).mean()
# Display the moving average
print(df[['10_period_ma']].tail())
This simple analysis will show the 10-period moving average of the stock price, which is helpful for identifying trends.
Step 5: Visualize the Data
If you want to visualize the stock price and moving averages, Python’s library can help:
bash
Copy code
pip install matplotlib
Then, you can plot the stock data like this:
python
Copy code
import matplotlib.pyplot as plt
# Plot the stock's closing price and moving average
plt.plot(df['4. close'].astype(float), label='Stock Price')
plt.plot(df['10_period_ma'], label='10 Period MA')
plt.title(f'{symbol} Stock Price with Moving Average')
plt.xlabel('Time')
plt.ylabel('Price (USD)')
plt.legend()
plt.show()
This will give you a clear visual representation of the stock’s performance and its moving average, making it easier to spot trends.
Best Practices for Using a Stock API Python
When working with a stock market API, it’s important to follow some best practices to make your code efficient and reliable:
-
Respect Rate Limits: Most APIs have rate limits for free plans. Make sure to check how many requests you can make per minute or day.
-
Store Data Locally: Storing data locally can save you time and API calls if you need to analyze the data later.
-
Error Handling: Always add error handling to your code. If something goes wrong with your API call, your program should not crash.
-
Secure Your API Key: Don’t hardcode your API key into your code. Use environment variables or configuration files to keep your key safe.
Conclusion
Integrating a stock API into your projects gives you powerful access to real-time and historical stock data. Whether you're building a stock tracker or analyzing market trends, a stock API Python will save you time and help you make informed decisions. With just a few lines of code, you can automate your stock market data collection, analyze trends, and even predict future stock movements.
FAQs
Q1: Do I need to pay for a stock API?
Most stock APIs offer free plans with limited features. For more advanced features or higher data limits, you may need to switch to a paid plan.
Q2: Can I track multiple stocks with one API?
Yes, most stock APIs allow you to request data for multiple stocks. You just need to change the stock symbol in the API request.
Q3: How do I handle large amounts of data?
If you’re dealing with large amounts of stock data, consider storing it in a database or using efficient data processing tools like pandas to manage and analyze the data.